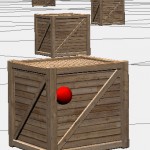
If you don’t come here from that previous post, here’s what this is about: When the user clicks somewhere on the screen we want to find the object in the scene that is actually being “clicked on”. More precise – we want to know when a user clicked on an imported COLLADA model. The most straight forward way to achieve this is to do ray casting.
I’ll be following along with this demo (it shows a couple of boxes and will set a marker to the point where you clicked on a box): https://jensarps.github.io/webgl_experiments/collada_picking_ray_r62.html
The annotated source is here: https://github.com/jensarps/webgl_experiments/blob/master/collada_picking_ray_r62.html
Differences to Revision 49
There’s two major good news for the current revision:
1. The performance and memory efficiency of three.js’ built-in ray casting classes have greatly improved.
2. The built-in raycaster is able to detect COLLADA models without any further modifications.
The main ideas behind the ReusableRay
class made it into three.js’ Raycaster
class. Effectively, that means that there is no more need for the ReusableRay
class that I recommended to use for prior revisions of three.js. Win!
If you compare the source code of the two collada_picking_ray versions on GitHub, you’ll see that it’s almost identical. Let’s go through the code again step by step; If there are changes to the previous versions, I’ll put a bold “Changes to R49:” below the code so that you can quickly skim through the post.
The Code
Initially, we setup some vars we will later need:
var raycaster = new THREE.Raycaster(); var projector = new THREE.Projector(); var directionVector = new THREE.Vector3();
The first thing we do is to record the mouse event. We do not react to it right now, because we don’t want to do anything outside of the render loop, so we keep control about what happens when.
Changes to R49: No more new ReusableRay()
– instead, we create a new instance of THREE.Raycaster
that we can re-use.
So let’s just store the coordinates and set a flag that we can later look up:
container.addEventListener('click', function (evt) { // The user has clicked; let's note this event // and the click's coordinates so that we can // react to it in the render loop clickInfo.userHasClicked = true; clickInfo.x = evt.clientX; clickInfo.y = evt.clientY; }, false);
Next, in the render loop, we check if a click has happened. If so, we start the whole ray casting thingy. To define the ray, we need two vectors: One representing the start point of the ray, and the other one representing the direction. The first is easy, it’s the camera position. The second is more interesting. We start with translating the mouse coordinates into something that’s independent of screen size, and assigning them to the direction vector:
// The following will translate the mouse coordinates into a number // ranging from -1 to 1, where // x == -1 && y == -1 means top-left, and // x == 1 && y == 1 means bottom right var x = ( clickInfo.x / SCREEN_WIDTH ) * 2 - 1; var y = -( clickInfo.y / SCREEN_HEIGHT ) * 2 + 1;
Now, there’s a little bit of math magic happening. Currently, we have the right direction if the camera’s position and view direction wouldn’t have changed. So we need to modify the vector to take these into account; I can’t really explain why this works, you need to trust me on this one:
// Unproject the vector projector.unprojectVector(directionVector, camera); // Substract the vector representing the camera position directionVector.sub(camera.position);
Changes to R49: Method name change: Vector3.subSelf()
now is Vector3.sub()
.
Ok, now we’ve got a vector that describes the direction correctly, but if you inspect it, you’ll find that it contains some crazy numbers. We need to make sure it contains only numbers ranging from -1 to 1 before passing it on to the ray caster class and firing off the ray:
// Normalize the vector, to avoid large numbers from the // projection and substraction directionVector.normalize(); // Now our direction vector holds the right numbers! raycaster.set(camera.position, directionVector);
Changes to R49: Like ReusableRay
, Raycaster
has a method to set the source and direction of the ray – and it takes the same arguments. So it’s an easy change: instead of ray.setSource()
, just call raycaster.set()
.
We can now ask the ray class for intersections, and it will report back all objects that intersect with our ray. Intersections are ordered by distance, so in this case, we only need the first one we get. The first argument is an array of objects that are possible candidates for an intersection. I recommend to optimize this, but for this example, I’m just passing all the scene’s children. If you have imported COLLADA models in your list of candidates, you need to set the second argument to “true”.
// Ask the raycaster for intersects with all objects in the scene: // (The second arguments means "recursive") var intersects = raycaster.intersectObjects(scene.children, true); if (intersects.length) { // intersections are, by default, ordered by distance, // so we only care for the first one. The intersection // object holds the intersection point, the face that's // been "hit" by the ray, and the object to which that // face belongs. We only care for the object itself. var target = intersects[0].object; statsNode.innerHTML = 'Name: ' + target.name + '<br>' + 'ID: ' + target.id; }
Changes to R49: The top level object of the imported COLLADA still is a plain Object3D
instance; but the new ray caster can dive down recursively into the objects and will find the mesh that the ray hit. To do this, the second parameter, “recursive” must be set to true
.
And that’s it – Mouse-picking COLLADA models has become really easy!