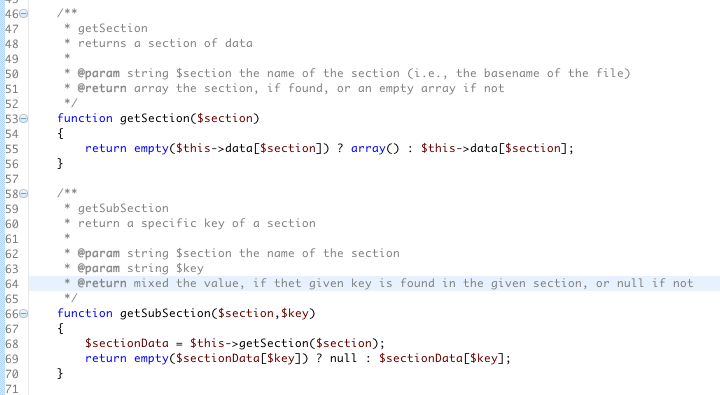
So I want to share today a very primitive static data handler that I use for quite some time now, and wich has always served me well. By static, I mean data that doesn’t change during runtime, like smtp server connection data, database connection data and the likes.
The idea is to have a seperate directory (named “static” in my case) with .data files in it. Every .data file should care about a certain aspect of static data needed in an application. This directory is then blocked via an .htaccess file, containing the single line:
# file: .htaccess Deny From All
During the bootsrap process of your app, the collector is called to walk through that directory and gather the data from all the files:
// file: bootstrap.php // load static data YourProject_StaticData::getInstance()->collectStaticData();
These files are pretty much standard config files, so they are readable via php’s function parse_ini_file. That function has two major limitations: It cannot handle hierarchy beyond two levels and it breaks when it encounters a quote character in a value. These limitations are discussed elsewhere, but with this static data collector we can at least push the first one a level further away. A data file could look like this:
# file: mailconfig.data [smtp] host = somehost.tld auth = true username = user@somehost.tld password = somepass [lists] whitelist = "goodone@example.com,admin@somehost.tld" blacklist = "badone@example.com" [options] checkwhitelist = false checkblacklist = true # ...
To get the connection data to the applications’s smtp account, you’d simply call:
$smtpConfig = YourProject_StaticData::getInstance()->getSubSection('mailconfig','smtp');
Fairly easy to use, pretty and readable. And it also is very handy for something that *very* often annoys me when looking into some client’s code: establishing a connection to a database. To different databases in most cases, for development, testing and deployment. I’ve seen pretty awkward attempts to handle this – but it can be done with one line of code, regardless of where the code is running. Say, you use Doctrine as ORM for your database, and have a data file like the following, using host names as keys (besides all my love for the NoSQL movement, I run into a mysql database on nearly every project):
# file: dbms.data project.local = "mysql://user:pass@localhost/project_db" test.project.tld = "mysql://testuser:testpass@project.tld/test_project_db" project.tld = "mysql://user:pass@project.tld/project_db"
you can easily connect to your database by defining a constant that contains the current host name (wich is a good idea, in general) and call
// file: bootstrap.php //set up a connection Doctrine_Manager::connection( YourProject_StaticData::getInstance()->getSubSection('dbms',HOST), 'mainConnection' )->setCharset("utf8");
Clean and short. And independant from your location – just see to it that your data file contains the right connection data for each host, and never touch that line of code in your bootstrapping process again.
The PHP class uses the singleton design pattern (as you already know from the code above), and is less than 100 lines of code in size. Including doc. It’s as simple as it is handy. And it can be easily extended – if the need arises, what never happened to me.
I hope you can find it as useful as I do!
You can grab the file over here: StaticData.php